Handling Forms In React
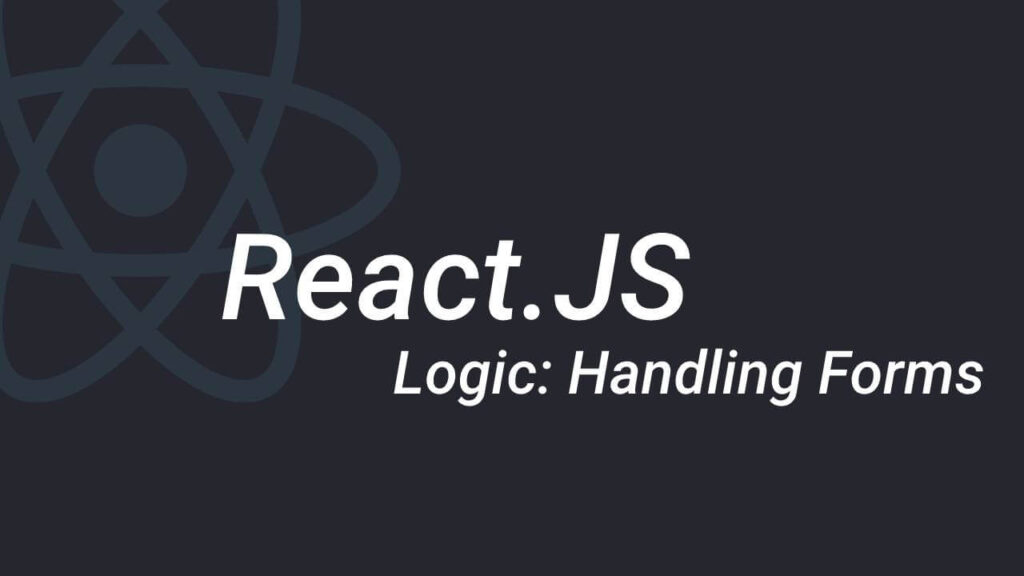
We can handle form in react and pass recive or pass thata from them.
For example We have an input form
// First We create a ref using
username = React.createRef(); // Class Components
const username = useRef(); // Functional Components
// We have a Input here
<input
ref={this.username} // class components
ref={username} // Functional Component
type="text"
name=""
id="username"
className="form-control"
/>
Then We can access data from input like
console.log(this.username.current.value); // Class components
console.log(username.current.value); // Functional components
Bonus Tip: If we want to focus on a input we can focus with
username.current.focus();
OR
<input type="text" autoFocus={true} />
Chnaging Input Data
We can change input data with defining handleChange function and get inout data from state
First we initial a state
// Class component
state = {
account: {
account : "",
password: "",
}
}
// Functional Component
const [account, setAccount] = useState({
username: "",
password: "",
});
// Class component
<input type="text" value={this.state.account.username} id="password" className="form-control" onChange={this.handleChange} />
// Functional Component
<input type="text" value={account.username} id="password" className="form-control" onChange={(e) => handleChange(e)} />
Also we add handle change function to handling state change
const handleChange = (e) => {
const accountClone = { ...account }; // this.state.account => Class component
accountClone.username = e.currentTarget.value;
setAccount(accountClone); // this.setState({ accountClone : account }) => Class component
};
Error Handling Issues
We can create a state and fill up the state like :
const [allErrors, setAllErrors] = useState();
// The validate function
const validate = (e) => {
const errors = {};
if (account.username.trim() === "") errors.username = "Username is empty";
if (account.password.trim() === "") errors.password = "Password is Empty";
return Object.keys(errors).length === 0 ? null : errors;
};
And in the submit function we can say:
const handleSubmit = (e) => {
e.preventDefault();
const errors = validate();
setAllErrors(errors || {});
if (errors) return;
};