Higher Order Components
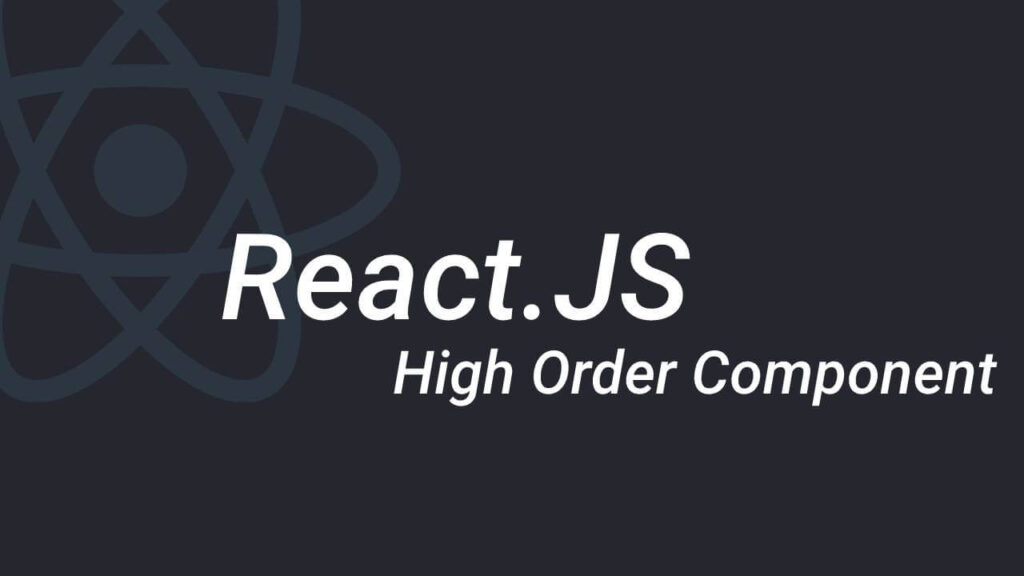
We use higher order components to reuse logic across components.
For creating a higher order component first we need a component that render a component as a child so something like this:
import React from "react";
function withTooltip(Component) {
return class WithTooltip extends React.Component {
state = { showTooltip: false };
mouseOver = () => this.setState({ showTooltip: true });
mouseOut = () => this.setState({ showTooltip: false });
render() {
return (
<div onMouseOver={this.mouseOver} onMouseOut={this.mouseOut}>
<Component {...this.props} showTooltip={this.state.showTooltip} />
</div>
);
}
};
}
export default withTooltip;
As you can see we have a function that returns a class component and as the return for the class component return, we return the given component.
And here in the component that we need to return with the reusable functionality we simply call the parent component as a function and give the current component as an argument like:
import React, { Component } from "react";
import withTooltip from "./withTooltip";
class Movies extends Component {
render() {
return <div>movies{this.props.showTooltip && "Hello"}</div>;
}
}
export default withTooltip(Movies); // Here we called it
In the end we call the Movies component in the app.js or where ever that we want it.
Passing Multiple Arguments
we can change the functionality of the HOC in the HOC with the arguments that we give to the HOC() function.
/* The New HOC Component */
import React, { Component } from "react";
const withCounter = (OldComponent, step) => {
class newComponent extends Component {
constructor() {
super();
this.state = {
number: 0,
};
}
clickHandler = () => {
this.setState((prevState) => ({ number: prevState.number + step }));
};
render() {
return <OldComponent number={this.state.number} clickHandler={this.clickHandler} />;
}
}
return newComponent;
};
export default withCounter;
/* Child of the HOC */
import React, { Component } from "react";
import HOCComponent from "./HOCComponent";
class ChildOfHOC extends Component {
render() {
return (
<div>
We have {this.props.number}
<button onClick={this.props.clickHandler}>Click Me to add 5</button>
</div>
);
}
}
export default HOCComponent(ChildOfHOC, 5); // Here we pass the second argument