How Use To Redux
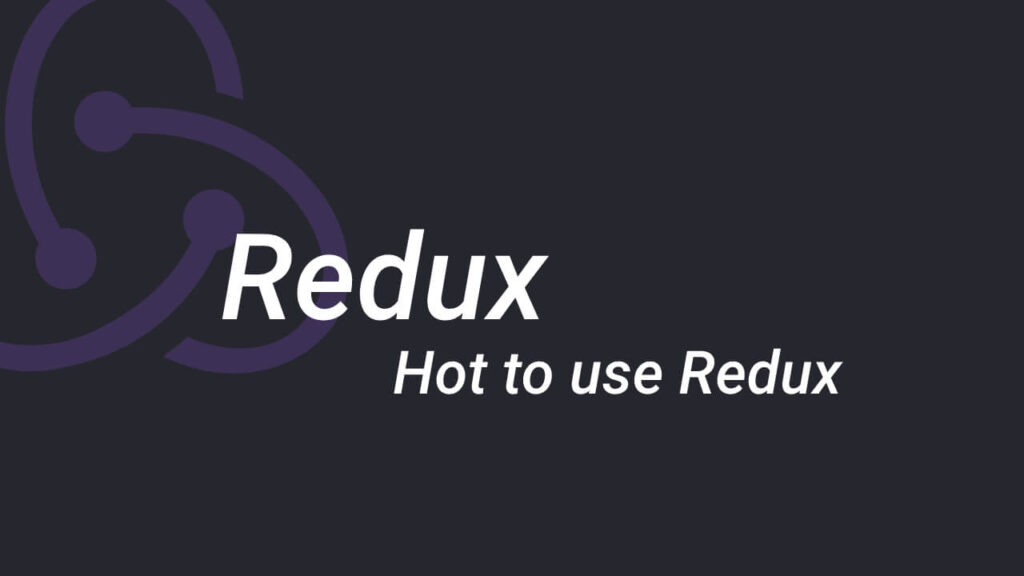
Here Lets Create A simple project using Redux and pass the Value to child using this tool.
First We need multiple files to handle this
- App.js
- ./components/Counter.js
- ./redux/counter/CounterAction.js
- ./redux/counter/CounterReduer.js
- ./redux/store.js
./redux/counter/CounterAction.js
Here we create a simple action type to call it in our switch statement in the CounterReducer.js from the component.
export const increase = () => {
return { type: "INCREASE" };
};
./redux/counter/CounterReducer.js
In this file we define a switch case to handle the action type. Same as useReducer in react!
const initialState = {
counter: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case "INCREASE":
return {
counter: state.counter + 1,
};
default:
return state;
}
};
export default counterReducer;
./redux/store.js
In this file we create a store ( the reducer provider ) to called it from the App.js.
import { createStore } from "@reduxjs/toolkit";
import counterReducer from "./counter/CounterReducer";
const store = createStore(counterReducer);
export default store;
App.js
in this file we simply call the store and provider to provide chuldren with the data same as the context API from react.
import { Provider } from "react-redux";
import Counter from "./components/Counter";
import store from "./redux/store";
function App() {
return (
<div className="App">
<Provider store={store}>
<Counter />
</Provider>
</div>
);
}
export default App;
./components/Counter.js Using map Functions
And in the counter.js component we call the connect method from the redux and connect Redux state and its action to the component to attach data to the PROPS of this component.
import React from "react";
import { connect } from "react-redux";
import { increase } from "../redux/counter/CounterAction";
{
/*------------------------------------*\
#Using Function to map to props
\*------------------------------------*/
}
const Counter = (props) => {
return (
<div>
Counter {props.counter}
<br />
<button onClick={props.increase}>Increase Me</button>
</div>
);
};
const stateProps = (state) => {
return {
counter: state.counter,
};
};
const actionProps = (dispatch) => {
return {
increase: () => dispatch(increase()),
};
};
export default connect(stateProps, actionProps)(Counter);
./components/Counter.js Using Hooks (Same As Top)
import React from "react";
import { useSelector, useDispatch } from "react-redux";
import { increase } from "../redux/counter/CounterAction";
{
/*------------------------------------*\
#Using Hooks
\*------------------------------------*/
}
const Counter = (props) => {
const counter = useSelector((state) => state.counter);
const dispatch = useDispatch();
return (
<div>
Counter {counter}
<br />
<button onClick={() => dispatch(increase())}>Increase Me</button>
</div>
);
};
export default Counter;