Redux Guidlines Part 2
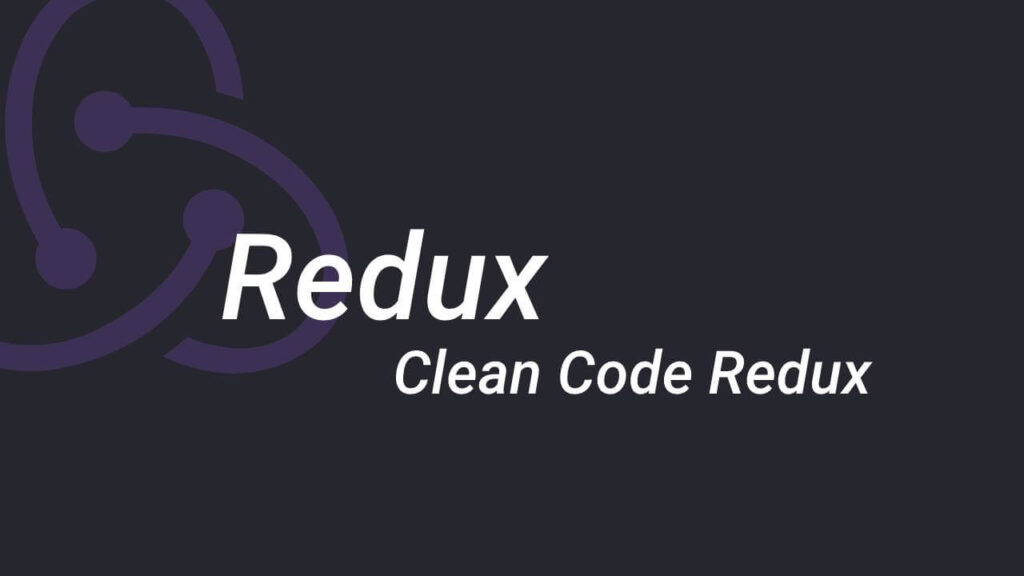
A better way to create a reducer using redux createSlice
/* bugs.js */
import { createSlice } from "@reduxjs/toolkit";
let lastId = 0;
const slice = createSlice({
name: "bugs",
initialState: [],
reducers: {
bugAdded: (bugs, action) => {
bugs.push({
id: lastId++,
description: action.payload.description,
resolved: false,
});
},
bugRemoved: (bugs, action) => {
bugs = bugs.filter((bug) => bug.id !== action.payload.id);
},
bugResolved: (bugs, action) => {
const index = bugs.findIndex((bug) => (bug.id === action.payload.id ? bug : { ...bug, resolved: true }));
},
},
});
export const { bugAdded, bugRemoved, bugResolved } = slice.actions;
export default slice.reducer;
For creating a store we can say
/** configureStore.js */
import { configureStore } from "@reduxjs/toolkit";
import reducer from "./bugs";
export default () => {
return configureStore({ reducer });
};
For creating actions we can use ducs pattern => /src/store/EXAMPLE.js
/** bugs.js OR EXAMPLE.JS */
import { createAction, createReducer } from "@reduxjs/toolkit";
// Action Creators
export const bugAdded = createAction("bugAdded");
export const bugRemoved = createAction("bugRemoved");
export const bugResolved = createAction("bugResolved");
// Reducer
let lastId = 0;
/**
*
* first index: initial state
*
* second argument: the reducer functionality
*
*/
export default createReducer([], {
// Dynamic type naming - top one is static
[bugResolved.type]: (bugs, action) => {
const index = bugs.findIndex((bug) => bug.id === action.payload.id);
bugs[index].resolved = true;
},
// bugs argument is the state (we can rename it)
bugAdded: (bugs, action) => {
bugs.push({
id: lastId++,
description: action.payload.description,
resolved: false,
});
},
[bugRemoved.type]: (bugs, action) => {
bugs = bugs.filter((item) => item.id === action.payload.id);
},
});
Finally in index.js we can use the store that we created
import configureStore from "./store/configureStore";
import { bugAdded } from "./store/bugs";
const store = configureStore();
store.dispatch(bugAdded({ description: "Desc Here" }));
Slices In Redux
For selecting data from redux store we can say
/* bugs.js */
export const getUnresolvedBugs = (state) => state.entities.bugs.filter((bug) => !bug.resolved);
/* index.js */
import { getUnresolvedBugs } from "./store/bugs";
const getunresolvedBugs = getUnresolvedBugs(store.getState());