@reduxjs/toolkit new methods
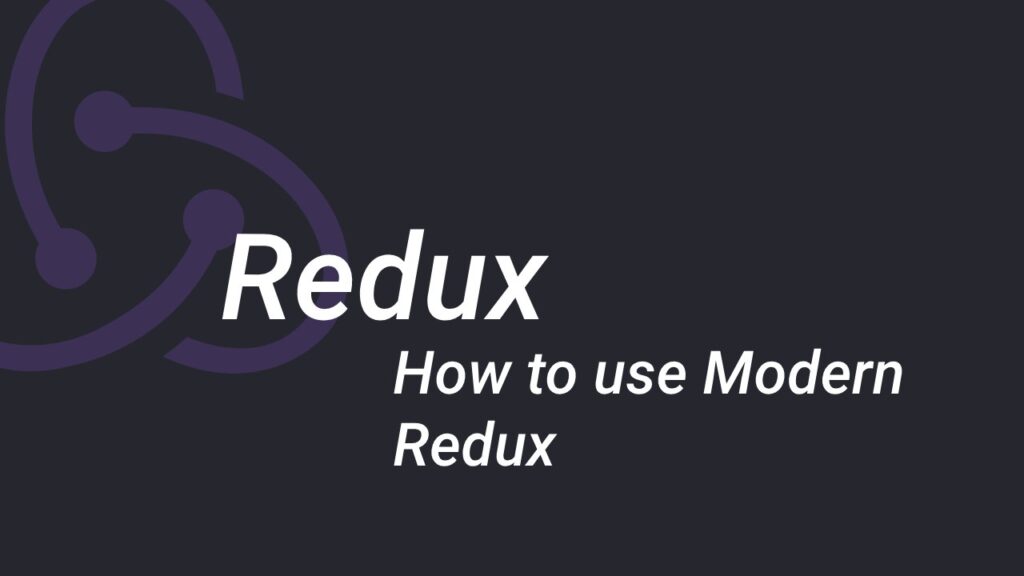
We can export types of the Rootstate and dispatch like this
export type RootState = ReturnType<typeof store.getState>
export type AppDispatch = typeof store.dispatch
Define Typed Hooks
We can create a hook that doesnt need that we import AppDispatch and RootState every time
import { useDispatch, useSelector } from 'react-redux'
import type { RootState, AppDispatch } from './store'
export const useAppDispatch = useDispatch.withTypes<AppDispatch>()
export const useAppSelector = useSelector.withTypes<RootState>()
We can define Payload type like this in the reducer
interface CounterState {
value: number
}
const initialState: CounterState = {
value: 0,
}
incrementByAmount: (state, action: PayloadAction<number>) => {
state.value += action.payload
},
RTKQ (Redux tool kit) – RTK QUery with redux
import { createApi, fetchBaseQuery } from '@reduxjs/toolkit/query/react'
import type { Pokemon } from './types'
export const pokemonApi = createApi({
reducerPath: 'pokemonApi',
baseQuery: fetchBaseQuery({ baseUrl: 'https://pokeapi.co/api/v2/' }),
endpoints: (builder) => ({
getPokemonByName: builder.query<Pokemon, string>({
query: (name) => `pokemon/${name}`,
}),
}),
})
export const { useGetPokemonByNameQuery } = pokemonApi
And then we should add it to the Store
import { configureStore } from '@reduxjs/toolkit'
import { setupListeners } from '@reduxjs/toolkit/query'
import { pokemonApi } from './services/pokemon'
export const store = configureStore({
reducer: {
[pokemonApi.reducerPath]: pokemonApi.reducer,
},
middleware: (getDefaultMiddleware) =>
getDefaultMiddleware().concat(pokemonApi.middleware),
})
setupListeners(store.dispatch)
Providing the store to the App level
import * as React from 'react'
import { render } from 'react-dom'
import { Provider } from 'react-redux'
import App from './App'
import { store } from './store'
const rootElement = document.getElementById('root')
render(
<Provider store={store}>
<App />
</Provider>,
rootElement
)
How to get data from the RTK Query
import * as React from 'react'
import { useGetPokemonByNameQuery } from './services/pokemon'
export default function App() {
const { data, error, isLoading } = useGetPokemonByNameQuery('bulbasaur')
return (
<div className="App">
{error ? (
<>Oh no, there was an error</>
) : isLoading ? (
<>Loading...</>
) : data ? (
<>
<h3>{data.species.name}</h3>
<img src={data.sprites.front_shiny} alt={data.species.name} />
</>
) : null}
</div>
)
}