TypeScript Basics
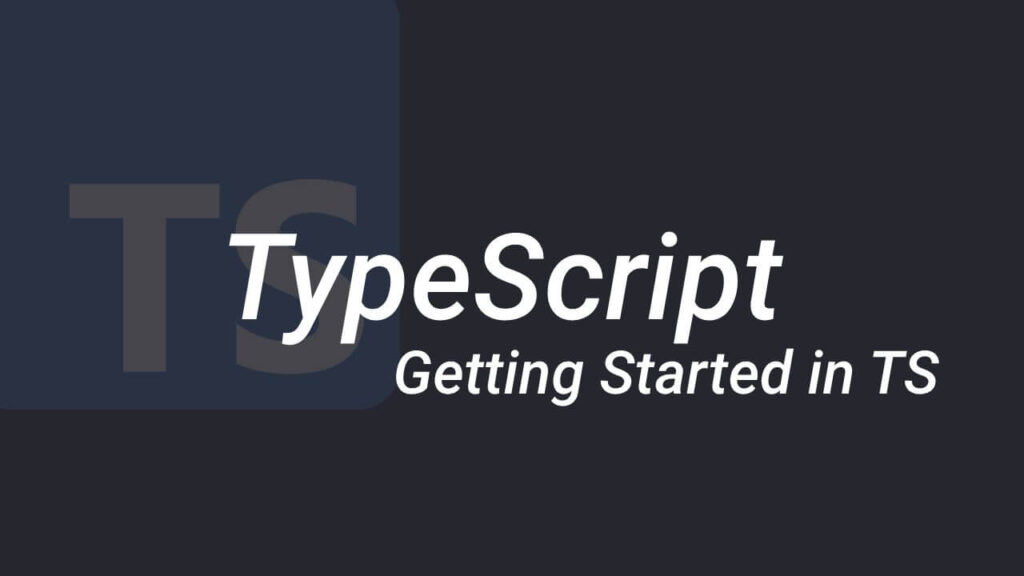
Defining a variable
let sales: number = 123_456; // Type number
let course: string = "String"; // Type string
let is_published = false; // Type boolean (We can dont mention the variable type)
let level; // Type Any
let numbers: number[] = [1, 2, 3]; // Define array values as numbers just numbers
let user: [number, string] = [1, "Nima"]; // Exactly match array length with values (tuples)
// enums PascalCase
const enum Size {
Small = 1,
Medium, // Automaticaly set 2
Large, // Automaticaly set 3
}
// Like this we can define own type and value
const myUser: Size = Size.Medium;
// Define object in ts
let employee: {
readonly id: number; // Read only value
name?: string; // optional value
retire: (date: Date) => void; // Function value
} = {
id: 1,
name: "Nima",
retire: () => {
console.log(Date);
},
};
// Giving instant, optional, default arguments
// the return before bracket will say function should return number
function calculateTax(income: number, taxYear?: number, taxYears = 2022): number {
if ((taxYear || 2022) < 50_000) return income * 1.2;
return income * 1.3 + taxYears;
}
TypeScript Config File
We can init ts config using this command
tsc --init
In the config file we can specify some thing for example we can change rendering options in tsconfig.json
"target": "ES2016" // Render version of javascript
"rootDir": "./src" // Root of the typescript files
"outDir": "./dist" // the .js files destination
"sourceMap": true // Create source map of rendered files
"removeComments": true // Remove comments :)
"noUnusedParameters": true // Raise an error when a function parameter isn't read
"noImplicitReturns": true, // Enable error reporting for codepaths that do not explicitly return in a function
"noUnusedLocals": true // give warning if local variable never used
"allowUnreachableCode": false, // Tell which code NOT running (using) never type
Type Aliases
We can define type aliases for the objects in TypeScript and make them reusable.
// difinition of type aliases (PasCal case)
type Employee = {
readonly id: number;
name?: string; // optional value
retire: (date: Date) => void;
};
// Using The Employee
let employee: Employee = {
id: 1,
name: "Nima",
retire: () => {
console.log(Date);
},
};
Union Type Checking
we can also define that variable or argument accept different type of types
// Union Type Checking
function kgToLbs(weight: number | string): number { // Accept number or string
if (typeof weight === "number") return weight * 2.2;//Intellisense detect number
else return parseInt(weight) * 2.2; // Intellisense detect that is string
}
Intersection types
Also we can define Intersection types and use them where ever that we want
// Intersection types
type Draggable = { // PasCal case
darg: () => void;
};
type Resizable = { // PasCal case
resize: () => void;
};
type UiWidgets = Draggable & Resizable; // Defining using (&)
let userWidget: UiWidgets = {
darg: () => {},// Using from Draggable
resize: () => {},// Using from Resizable
};
Literal Types
We can define static values for variables using literal types they accept numbers and strings
// Literal types
type Quantity = 50 | 100; // We can assign just 50 or 100
let quantity: Quantity = 50; // Defining a quantity type
// Using String type
type Metric = "cm" | "inch";
Optional Chaining
We can detect if argument is not null and not undefined just like this code. Just using the (?) mark we can define that if value is not null or undefined
// Optional Chaining
type Customer = {
birthDay: Date;
userId: number; // Just Here chilling
};
function getCustomer(id: number): Customer | null {
return id === 0 ? null : { birthDay: new Date(), userId: 0 }; // Should be two of them (birthDay & userId)
}
let customer = getCustomer(2);
// (customer?) will say if (customer) is NOT null and is NOT undefined
console.log(customer?.birthDay);
// (customer?.birthDay?) will say if both of them NOT null and is NOT undefined
console.log(customer?.birthDay?.getFullYear());
let log: any = null;
log?.("a"); // Will execute if it reference a function
Nullish Operator
We can check if given value is null (NOT ZERO => because zero(0) is a falsy value in JS) or it has value
let speed: number | null = -2;
let ride = {
// Nullish Operator
// (??) will say if value was true put there (speed) otherwise put 30
speed: speed ?? 30,
};
console.log(ride); // if null give 30 if (0) give (0) if (other value) give (other value)
Type Assertion
We can define that we know more about given element to TypeScript. For example TypeScript by default doesn’t know that given html element is input or not and because of that it doesn’t give us the (VALUE) property.
let address = document.getElementById("address");
let phone = document.getElementById("phone") as HTMLInputElement; // We say "Hey TypeScript" we know it is a input value element
let phone_2 = <HTMLInputElement>document.getElementById("phone"); // Other Convension of top code
console.log(address); // Doesnt Have (VALUE) property
console.log(phone.value); // Have (VALUE) property { If element as undefined program will crash }
Unknown Type
We can use unknown type for some arguments and values it give us better type checking than any type. (any) type accept everything and it is not good in TypeScript.
// Using (unknown) is preferred instead of any (Give us better type checking)
function render(document: any) {
// This is equal to if(typeof document === 'string').
// But with instance of we can say custom objet
if (document instanceof WordDocument) { // WordDocument is (type) for example
document.toUpperCase();
}
// type of just work for `string` , `boolean` , `number`
if (typeof document === "string") {
document.toUpperCase();
}
}
Never Type
When use never type to a function we say to TypeScript that this function never returns
function processEvent(): never {
while (true) {
// Infinite Loop (Never Returns)
}
}
processEvent();
console.log("hello World");