Useful JS Tips you should know
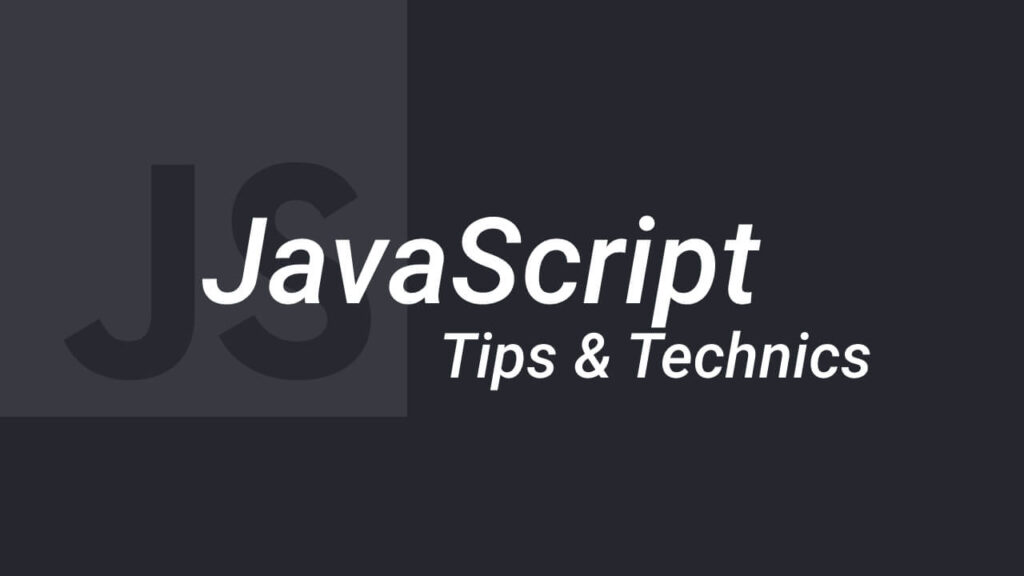
In this article, we will look at some useful methods that we can use in JS to reduce our code and make our lives easier 🙂
Spreading Objects
We can spread objects using ( … ) to spread objects and combine them together.
const first = { name: "Nima" };
const second = { job: "Front-End" };
const combined = { ...first, ...second, location: "DE" };
Spreading Arrays
Like the last code snippet, Also here we can use the same logic
const first = [1, 2, 3];
const second = [4, 5, 6];
const combined = [...first, "A1", ...second, "A2"];
Calling and Changing Children of an Object
We can call and change children of objects very easily like:
const person = {
name: "Nima",
walk() {}, // New method
talk: function () {}, // Old method
};
person.talk(); // Simple calling method from an object
person['name'] = 'john'; // Simply changing the value of children of an object
person.name = 'john'; // We can also access children of an object like this
const targetMember = 'NAME';
person[targetMember] = 'NEW NAME'; // Dynamically access an object using a variable or whatever :)
This Key word
This keyword in JS acts differently from other programming languages. See The example below.
const person = {
name: "Nima",
walk() {
console.log(this); // (this) here will refer to the person object
},
};
person.walk(); // Will refer to person Object
const walk = person.walk; // Refrecing walk method to variable NOT calling it
walk(); // HERE (this) keyword return undefined (Restrict Mode) and point to window Object :|
Bind in JS
Everything in JS is object even functions. We can use the bind keyword to bind a function to an object
const person = {
name: "Nima",
walk() {
console.log(this);
},
};
person.walk();
const walk = person.walk.bind(person); // Binding walk to person Object
walk(); // No Longer Window object (Undefined in Restricted Mode)
Arrow Functions
We can use arrow functions and their abilities to reduce our code with other benefits
const square = (number) => number * number; // Simple example
console.log(square(5)); // Code is shorter as you can see
const jobs = [
{ id: 1, isActive: true },
{ id: 2, isActive: true },
{ id: 3, isActive: false },
];
const activeJobs = jobs.filter((job) => job.isActive); // Return jobs that they are active :)
Arrow Functions and THIS keyword
arrow functions bind this keyword automatically or more accurate not re-bind This 😛
const person = {
talk() {
setTimeout(function () {
console.log("this", this); // Will Point to window Object (Because of OLD function keyword)
}, 800);
setTimeout(() => {
console.log("this", this); // Will point to Person Object (using arrow function)
}, 1000);
},
};
Array Map
Array.map iterate inside items of the array with given callback
const colors = ["red", "green", "blue"];
const items = colors.map((color) => `<li>${color}</li>`); // Using ( ` ` ) we can create template literal
// items variable will return list of colors :)
Objects Destructuring
We can destruct objects and reduce our code like example below
const address = {
street: "",
city: "",
country: "",
};
// UGLY UGLUUU UGLY :P
const street = address.street;
const city = address.city;
const country = address.country;
// Clean Code is here :D
const { street: st, city, country } = address; // Also We can use ( : ) to define another name for item
Classes In JS
We can define classes and use theme multiple times link example below
class Person {
constructor(name) {
this.name = name;
}
walk() {
console.log("walk", this.name);
}
}
const person = new Person("nima");
person.walk(); // Display Nima :) - Using this implementation we can reduce coding and make maintenance easier
Classes Inheritance
We can inherit parent methods and values from the parent class to the children. See example below
class Person {
constructor(name) {
this.name = name;
}
walk() {
console.log("walk", this.name);
}
}
class Teacher extends Person {
// Here using ( extends ) we can inherit methods from parent class :)
constructor(name, degree) {
super(name); // Using ( super ) we can overwrite parents arguments -> "name" is overwritted here
this.degree = degree;
}
teach() {
console.log("teach");
}
}
const codeTeacher = new Teacher("Mosh", "MSC"); // Teachers Should walk as well :)
Modules Creation
Also, we can define modules to reduce our code size by putting classes into an external file (Like CSS style sheets) and import them where ever that we want them
/******** Our index.js ***********/
import { Teacher } from "./teacher"; // Location of teacher module
const codeTeacher = new Teacher("Mosh", "MSC");
codeTeacher.teach();
/******** Our teacher.js ***********/
import { Person } from "./person"; // Location of person module
export class Teacher extends Person {
constructor(name, degree) {
super(name);
this.degree = degree;
}
teach() {
console.log("teach");
}
}
/******** Our person.js ***********/
export class Person {
constructor(name) {
this.name = name;
}
walk() {
console.log("walk", this.name);
}
}
* Note that it is important to export modules from other files.
Named Default Exports
We can define multiple exports for a module and also define which one is the default one. Look at the example below:
/******** Our teacher.js ***********/
import { Person } from "./person";
// Normal Export is here
export function handleDegree() {
/* Some JS Code Here */
}
// Default Export is here
export default class Teacher extends Person {
constructor(name, degree) {
super(name);
this.degree = degree;
}
teach() {
console.log("teach");
}
}
/******** Our index.js ***********/
import Teacher, { handleDegree } from "./teacher";
// We can import the default one (without parentheses) and the normal one in parentheses