Using Axios In React
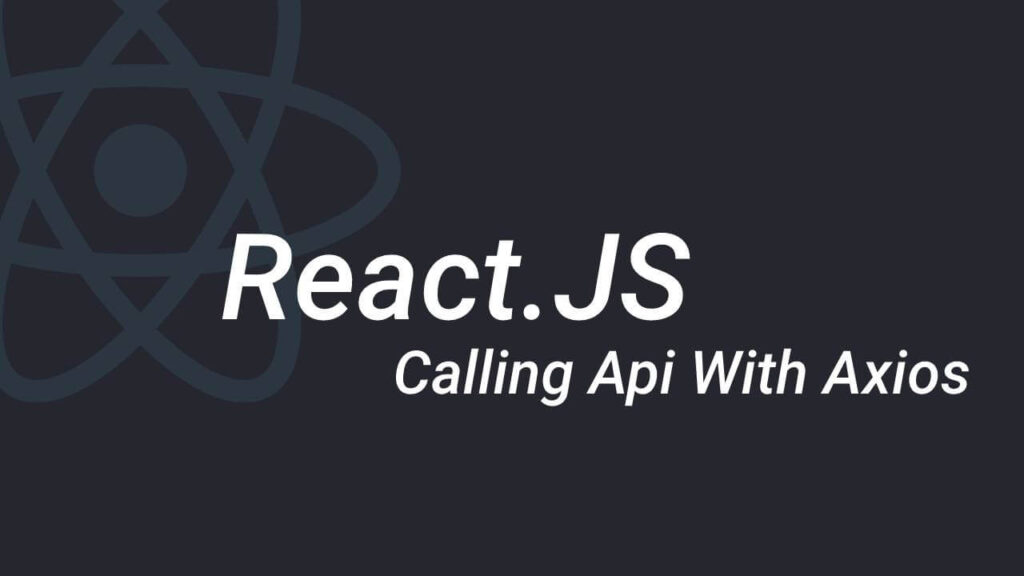
In this tutorial, we are going to fetch data intro react using Axios and see how is working under the hood. Also, we take a simple look at the proper definition of Class components and Functional components.
First we install Axios using:
npm i axios
GET Request
The create a get method using promise. A promise is an object that holds the result of an asynchronous operation. Async is an operation that completes in future
const promis = axios.get("https://jsonplaceholder.typicode.com/posts");
After that, the promise will be either resolved / fulfilled (success) or rejected (failed). Also Headers are a piece of information that the server sends to the client
Always We have to put the data fetching in the mount life cycle / useEffect in react and make it Async function and await the process that we need it from a promise. Look at the code below: (GET)
// Class Component
async componentDidMount() {
const response = await axios.get("https://jsonplaceholder.typicode.com/posts");
console.log(response);
}
// Functional Components
useEffect(() => {
async function getUsers() {
const result = await axios.get("URL");
setUsers(result.data); // useState
}
getUsers();
});
POST Request
For Sending data to the server also we can say: (POST)
handleAdd = async () => {
const obj = { title: "a", body: "b" };
const { data: post } = await axios.post(apiEndPoint, obj);
const posts = [post, ...this.state.posts];
this.setState({ posts }); // Class Component
setUsers(posts); // Functional Component
};
PUT Request
We can Also update data using axios.put (PUT):
handleUpdate = async (post) => {
post.title = "Updated";
await axios.put(apiEndPoint + "/" + post.id, post);
const posts = [...this.state.posts];
const index = posts.indexOf(post);
posts[index] = { ...post };
this.setState({ posts }); // Class Component
setUsers(posts); // Functional Component
};
DELETE Request
We can delete data by using axios: (DELETE)
handleDelete = async (post) => {
const originPosts = this.state.posts; // Cloning state
const posts = this.state.posts.filter((p) => p.id !== post.id);
this.setState({ posts });
try {
await axios.delete(`${apiEndPoint}/${post.id}`);
throw new Error("");
} catch (ex) {
alert("Updating Has failed");
this.setState({ posts: originPosts });
}
};
Type of errors in API Request
Expected Errors ( 404: Not Found (Page not found) , 400: Bad Request (Invalid given data) )
- Display Specific Error message to client
Unexpected Errors (Network down, server down, DB down, bug)
- Log Them (Errors)
- Display Generic and friendly message to client