Using PropTypes in React
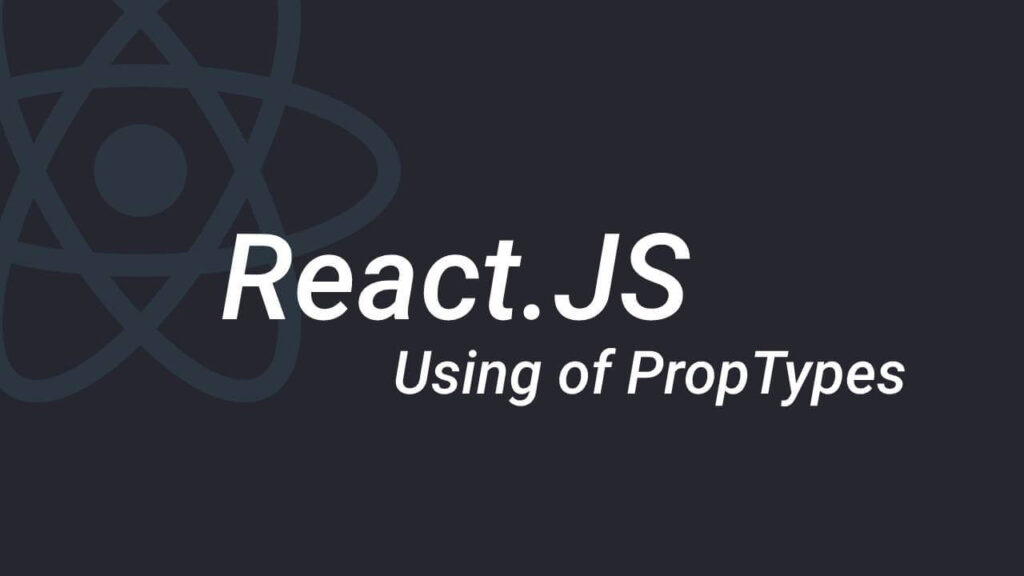
One of the most important tools to check the prop types in react is the prop-types library. In this article we are going to create a simple prop type using this library.
First of all try install propTypes in your project.
npm i prop-types
Imagine that we have a component that will get few props as properties something like code below
const Pagination = (props) => {
const { itemsCount, pageSize, currentPage, onPageChange } = props;
return (
<h1>Simple Component</h1>
);
};
So in this component we are getting the props. First for using prop types we should import it on the top
import PropTypes from "prop-types";
And just before exporting component we should use the prop types library and assign values to it.
Pagination.propTypes = {
itemsCount: PropTypes.number.isRequired, // Expect Required Number
pageSize: PropTypes.number.isRequired, // Expect Required Number
currentPage: PropTypes.number.isRequired, // Expect Required Number
onPageChange: PropTypes.func.isRequired, // Expect Required Function
};
export default Pagination;
For other prop types you can visit https://www.npmjs.com/package/prop-types
Hope it was helpful. Please comment your thoughts.