what is useEffect
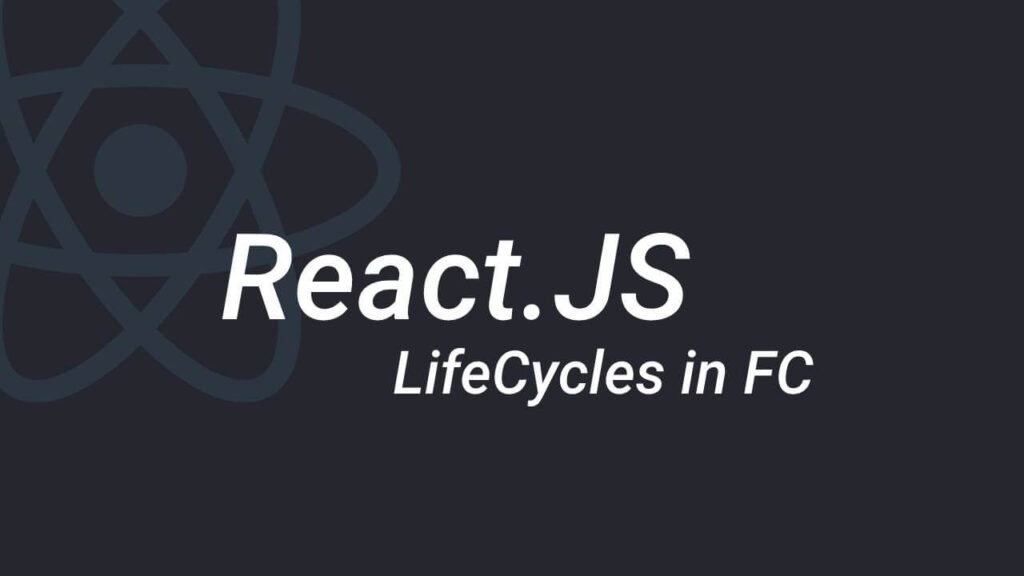
The useEffect Hook allows you to perform side effects in your components. In class components we have life cycle hooks like componentDidMount, componentDidUpdate and other hooks but in the functional components we have the useEffect see some examples below
componentDidMount
// Every time that component builds or change this code run
useEffect(() => {
document.title = "Test Title";
});
componentDidUpdate
// Every time that VARIABLE changes the useEffect will run
useEffect(() => {
document.title = "Test Title";
}, [VARIABLE]);
componentWillUnmount
// It act like componentDidUnmount (With using []) in the useEffect
useEffect(() => {
return () => {
// Code Here
};
}, []);
Fetching Data using useEffect
Also if we want to make a fetch / Axios call we can use useEffect hook to achieve this
// Making a fetch call using axios in useEffect
useEffect(() => {
async function getUsers() {
const result = await axios.get("URL"); // Getting data from API
setUsers(result.data); // State hook
}
});